How to Design a Custom Loan Calculator in Framer: Step-by-Step Guide
How to Design a Custom Loan Calculator in Framer: Step-by-Step Guide
How to Design a Custom Loan Calculator in Framer: Step-by-Step Guide
How to Design a Custom Loan Calculator in Framer: Step-by-Step Guide
6 minutes read
6 minutes read
4th Jan, 2025
4th Jan, 2025
Learn how to design a dynamic loan calculator in Framer with real-time calculations. Follow this step-by-step guide to enhance user experience and functionality.
Learn how to design a dynamic loan calculator in Framer with real-time calculations. Follow this step-by-step guide to enhance user experience and functionality.
Imagine this: You’re building a sleek custom loan calculator for a client. They’ve sent you the Figma files, you’ve integrated the calculator using Framer’s native form tools, but there’s a problem. The values won’t update dynamically when users select the loan amount, interest rate, or number of days. Sound familiar?
This blog post will walk you through creating a fully functional loan calculator in Framer. Let’s unravel this challenge together!
Imagine this: You’re building a sleek custom loan calculator for a client. They’ve sent you the Figma files, you’ve integrated the calculator using Framer’s native form tools, but there’s a problem. The values won’t update dynamically when users select the loan amount, interest rate, or number of days. Sound familiar?
This blog post will walk you through creating a fully functional loan calculator in Framer. Let’s unravel this challenge together!
Imagine this: You’re building a sleek custom loan calculator for a client. They’ve sent you the Figma files, you’ve integrated the calculator using Framer’s native form tools, but there’s a problem. The values won’t update dynamically when users select the loan amount, interest rate, or number of days. Sound familiar?
This blog post will walk you through creating a fully functional loan calculator in Framer. Let’s unravel this challenge together!
Why Does a Custom Loan Calculator Matter?
Why Does a Custom Loan Calculator Matter?
A loan calculator isn’t just a tool; it’s a powerful way to enhance user experience and drive conversions. Visitors can quickly estimate their financial commitments, making your product or service more accessible and engaging. However, ensuring its functionality is vital for maintaining user trust and satisfaction.
Common Challenges Developers Face:
Dynamic Updates: Ensuring values refresh in real-time when users adjust inputs.
Complex Calculations: Managing interdependent variables like loan amount, interest rate, and duration.
Integration Issues: Adapting designs from Figma files seamlessly into Framer.
A loan calculator isn’t just a tool; it’s a powerful way to enhance user experience and drive conversions. Visitors can quickly estimate their financial commitments, making your product or service more accessible and engaging. However, ensuring its functionality is vital for maintaining user trust and satisfaction.
Common Challenges Developers Face:
Dynamic Updates: Ensuring values refresh in real-time when users adjust inputs.
Complex Calculations: Managing interdependent variables like loan amount, interest rate, and duration.
Integration Issues: Adapting designs from Figma files seamlessly into Framer.
A loan calculator isn’t just a tool; it’s a powerful way to enhance user experience and drive conversions. Visitors can quickly estimate their financial commitments, making your product or service more accessible and engaging. However, ensuring its functionality is vital for maintaining user trust and satisfaction.
Common Challenges Developers Face:
Dynamic Updates: Ensuring values refresh in real-time when users adjust inputs.
Complex Calculations: Managing interdependent variables like loan amount, interest rate, and duration.
Integration Issues: Adapting designs from Figma files seamlessly into Framer.
Step-by-Step Solution to Build a Custom Loan Calculator
Step-by-Step Solution to Build a Custom Loan Calculator
Step 1: Import and Setup Your Figma Design
Export Figma Components: Ensure your design is cleanly exported into Framer.
Organize Layers: Group elements logically, such as input fields for the loan amount, interest rate, and number of days.
Use Native Form Elements in Framer: Replace static fields with Framer’s native form components to enable user interaction.
Step 2: Add Input Fields and Labels for Loan Amount, Interest Rate, and Duration
Loan Amount: Create a number input field.
Interest Rate: Add a percentage slider or another input for this value.
Number of Days: Use a dropdown or text field for duration selection.
Step 3: Implement Real-Time Calculations for Loan Amount and Interest Rate
Use Framer’s code overrides to handle dynamic updates:
import React, { useState } from "react"; export function LoanCalculatorOverride() { const [loanAmount, setLoanAmount] = useState(0); const [interestRate, setInterestRate] = useState(0); const [days, setDays] = useState(0); const [total, setTotal] = useState(0); function calculateTotal() { const dailyRate = interestRate / 365; const interest = loanAmount * dailyRate * days; setTotal(loanAmount + interest); } return ( <div> <input type="number" placeholder="Loan Amount" onChange={(e) => { setLoanAmount(parseFloat(e.target.value) || 0); calculateTotal(); }} /> <input type="number" placeholder="Interest Rate" onChange={(e) => { setInterestRate(parseFloat(e.target.value) || 0); calculateTotal(); }} /> <input type="number" placeholder="Days" onChange={(e) => { setDays(parseInt(e.target.value) || 0); calculateTotal(); }} /> <div>Total: {total}</div> </div> ); }
Attach this override to your input fields and total display to ensure real-time updates.
Step 4: Debug Common Issues in Your Custom Loan Calculator
Values Not Updating? Check your event listeners.
Calculations Wrong? Verify formulas and test edge cases like zero or extremely high values.
Styling Off? Align with your Figma design to maintain consistency.
Step 5: Test Responsiveness of Your Loan Calculator
Test your calculator on various devices and browsers to ensure flawless performance across platforms.
Step 1: Import and Setup Your Figma Design
Export Figma Components: Ensure your design is cleanly exported into Framer.
Organize Layers: Group elements logically, such as input fields for the loan amount, interest rate, and number of days.
Use Native Form Elements in Framer: Replace static fields with Framer’s native form components to enable user interaction.
Step 2: Add Input Fields and Labels for Loan Amount, Interest Rate, and Duration
Loan Amount: Create a number input field.
Interest Rate: Add a percentage slider or another input for this value.
Number of Days: Use a dropdown or text field for duration selection.
Step 3: Implement Real-Time Calculations for Loan Amount and Interest Rate
Use Framer’s code overrides to handle dynamic updates:
import React, { useState } from "react"; export function LoanCalculatorOverride() { const [loanAmount, setLoanAmount] = useState(0); const [interestRate, setInterestRate] = useState(0); const [days, setDays] = useState(0); const [total, setTotal] = useState(0); function calculateTotal() { const dailyRate = interestRate / 365; const interest = loanAmount * dailyRate * days; setTotal(loanAmount + interest); } return ( <div> <input type="number" placeholder="Loan Amount" onChange={(e) => { setLoanAmount(parseFloat(e.target.value) || 0); calculateTotal(); }} /> <input type="number" placeholder="Interest Rate" onChange={(e) => { setInterestRate(parseFloat(e.target.value) || 0); calculateTotal(); }} /> <input type="number" placeholder="Days" onChange={(e) => { setDays(parseInt(e.target.value) || 0); calculateTotal(); }} /> <div>Total: {total}</div> </div> ); }
Attach this override to your input fields and total display to ensure real-time updates.
Step 4: Debug Common Issues in Your Custom Loan Calculator
Values Not Updating? Check your event listeners.
Calculations Wrong? Verify formulas and test edge cases like zero or extremely high values.
Styling Off? Align with your Figma design to maintain consistency.
Step 5: Test Responsiveness of Your Loan Calculator
Test your calculator on various devices and browsers to ensure flawless performance across platforms.
Step 1: Import and Setup Your Figma Design
Export Figma Components: Ensure your design is cleanly exported into Framer.
Organize Layers: Group elements logically, such as input fields for the loan amount, interest rate, and number of days.
Use Native Form Elements in Framer: Replace static fields with Framer’s native form components to enable user interaction.
Step 2: Add Input Fields and Labels for Loan Amount, Interest Rate, and Duration
Loan Amount: Create a number input field.
Interest Rate: Add a percentage slider or another input for this value.
Number of Days: Use a dropdown or text field for duration selection.
Step 3: Implement Real-Time Calculations for Loan Amount and Interest Rate
Use Framer’s code overrides to handle dynamic updates:
import React, { useState } from "react"; export function LoanCalculatorOverride() { const [loanAmount, setLoanAmount] = useState(0); const [interestRate, setInterestRate] = useState(0); const [days, setDays] = useState(0); const [total, setTotal] = useState(0); function calculateTotal() { const dailyRate = interestRate / 365; const interest = loanAmount * dailyRate * days; setTotal(loanAmount + interest); } return ( <div> <input type="number" placeholder="Loan Amount" onChange={(e) => { setLoanAmount(parseFloat(e.target.value) || 0); calculateTotal(); }} /> <input type="number" placeholder="Interest Rate" onChange={(e) => { setInterestRate(parseFloat(e.target.value) || 0); calculateTotal(); }} /> <input type="number" placeholder="Days" onChange={(e) => { setDays(parseInt(e.target.value) || 0); calculateTotal(); }} /> <div>Total: {total}</div> </div> ); }
Attach this override to your input fields and total display to ensure real-time updates.
Step 4: Debug Common Issues in Your Custom Loan Calculator
Values Not Updating? Check your event listeners.
Calculations Wrong? Verify formulas and test edge cases like zero or extremely high values.
Styling Off? Align with your Figma design to maintain consistency.
Step 5: Test Responsiveness of Your Loan Calculator
Test your calculator on various devices and browsers to ensure flawless performance across platforms.
Key Takeaways for Designing a Loan Calculator in Framer
Key Takeaways for Designing a Loan Calculator in Framer
Custom Loan Calculator: Build a user-friendly, dynamic tool that enhances user trust.
Framer Native Form: Leverage this feature for seamless interaction.
Dynamic Calculations: Use code overrides to ensure real-time updates.
Align Design & Functionality: Transition smoothly from Figma to Framer without losing design integrity.
Custom Loan Calculator: Build a user-friendly, dynamic tool that enhances user trust.
Framer Native Form: Leverage this feature for seamless interaction.
Dynamic Calculations: Use code overrides to ensure real-time updates.
Align Design & Functionality: Transition smoothly from Figma to Framer without losing design integrity.
Custom Loan Calculator: Build a user-friendly, dynamic tool that enhances user trust.
Framer Native Form: Leverage this feature for seamless interaction.
Dynamic Calculations: Use code overrides to ensure real-time updates.
Align Design & Functionality: Transition smoothly from Figma to Framer without losing design integrity.
Let’s Build Together!
Let’s Build Together!
Creating a custom loan calculator in Framer may seem daunting, but with the right steps, it’s entirely achievable. Follow the guide above, and you’ll deliver a powerful tool that makes a real impact.
Have a question or want to share your experience? Drop a comment below or connect with us to explore more design and development insights.
Ready to create stunning, functional tools in Framer? Start now and take your designs to the next level!
Creating a custom loan calculator in Framer may seem daunting, but with the right steps, it’s entirely achievable. Follow the guide above, and you’ll deliver a powerful tool that makes a real impact.
Have a question or want to share your experience? Drop a comment below or connect with us to explore more design and development insights.
Ready to create stunning, functional tools in Framer? Start now and take your designs to the next level!
Creating a custom loan calculator in Framer may seem daunting, but with the right steps, it’s entirely achievable. Follow the guide above, and you’ll deliver a powerful tool that makes a real impact.
Have a question or want to share your experience? Drop a comment below or connect with us to explore more design and development insights.
Ready to create stunning, functional tools in Framer? Start now and take your designs to the next level!

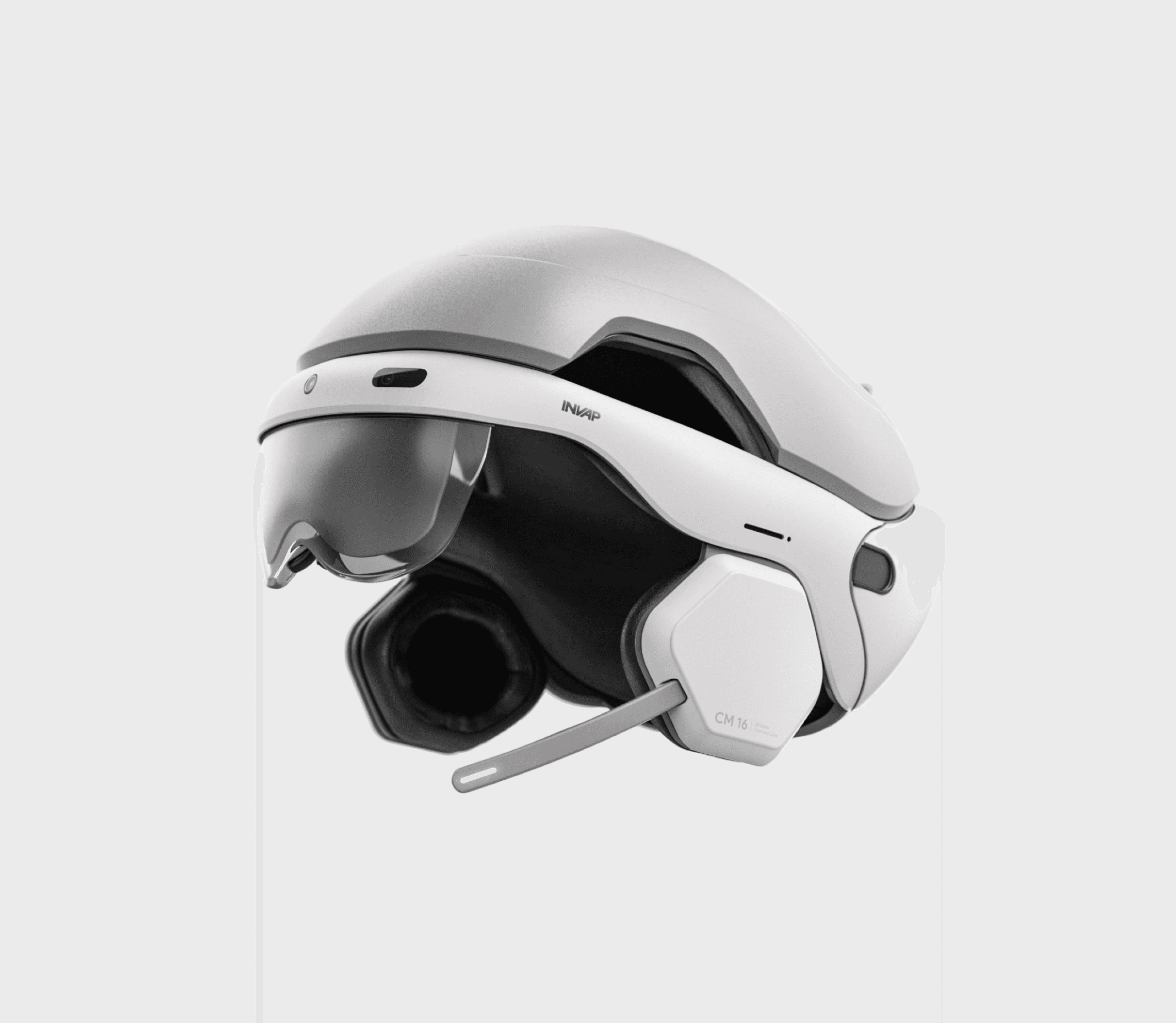
10th Jan 2025

Local SEO Strategies for 2025: Outpace Your Competitors with These Proven Tactics

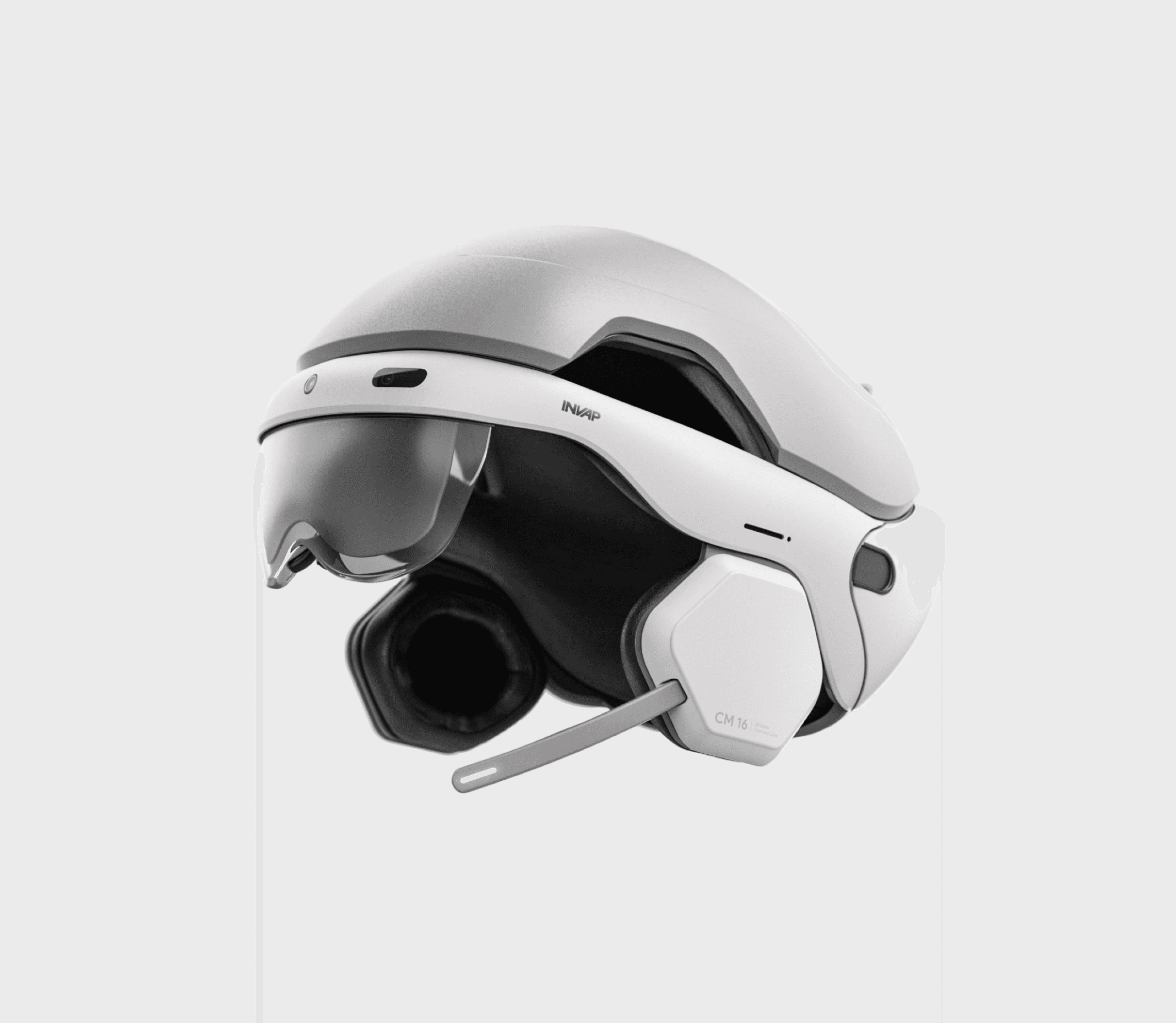
10th Jan 2025

Local SEO Strategies for 2025: Outpace Your Competitors with These Proven Tactics

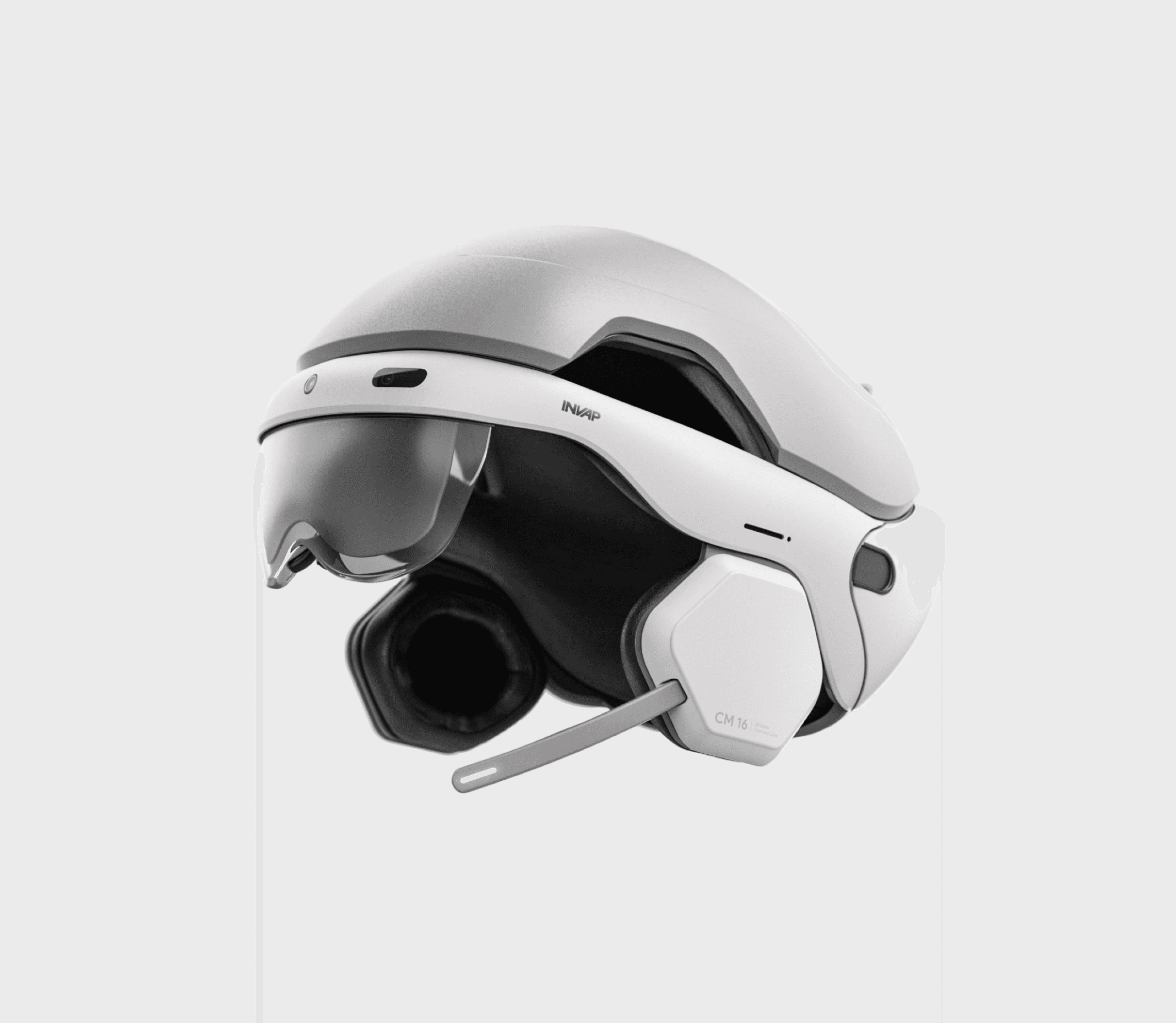
10th Jan 2025

Local SEO Strategies for 2025: Outpace Your Competitors with These Proven Tactics

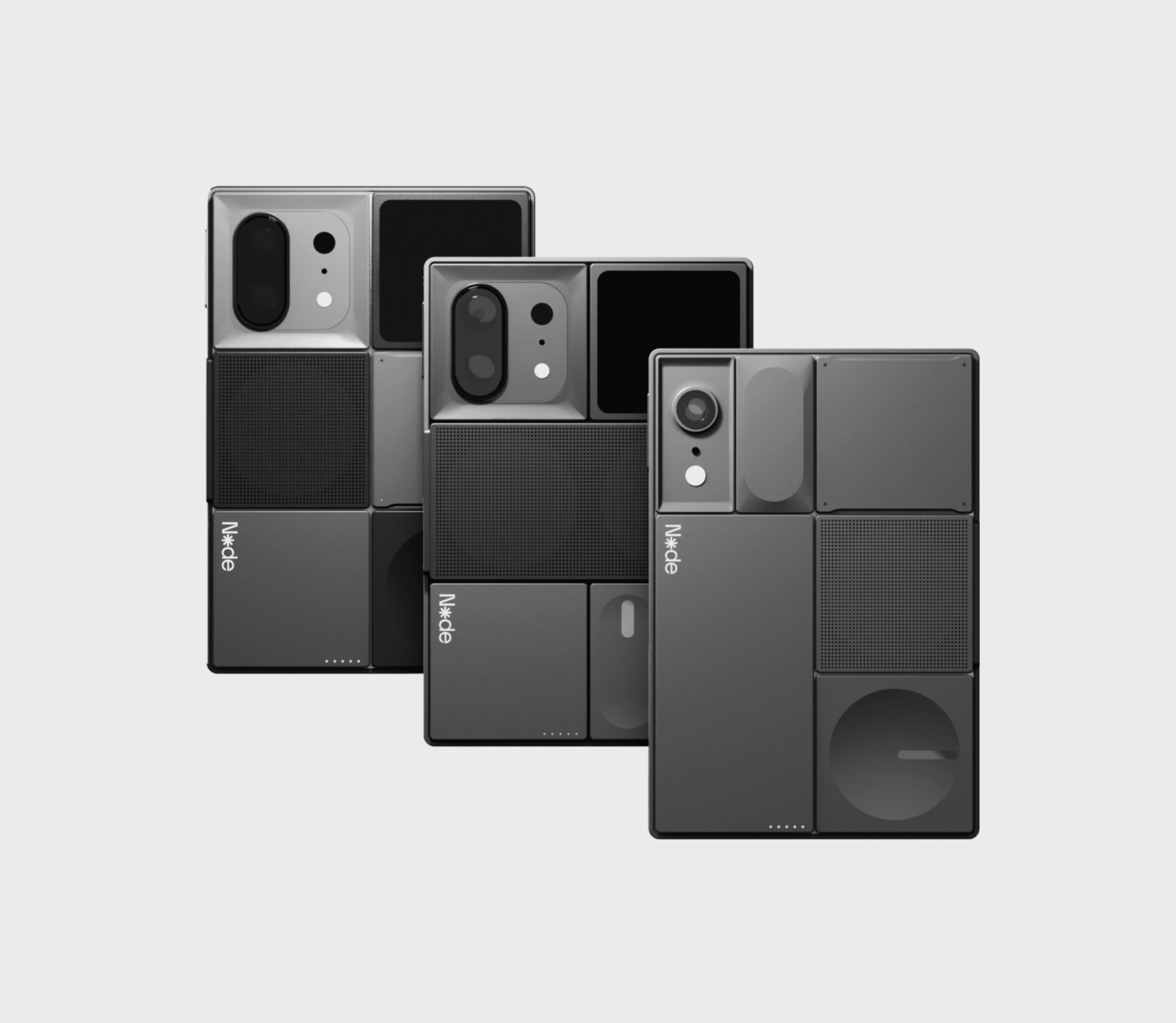
8th Jan, 2025

What Does a Perfect On-Page SEO Landing Page Structure Look Like?

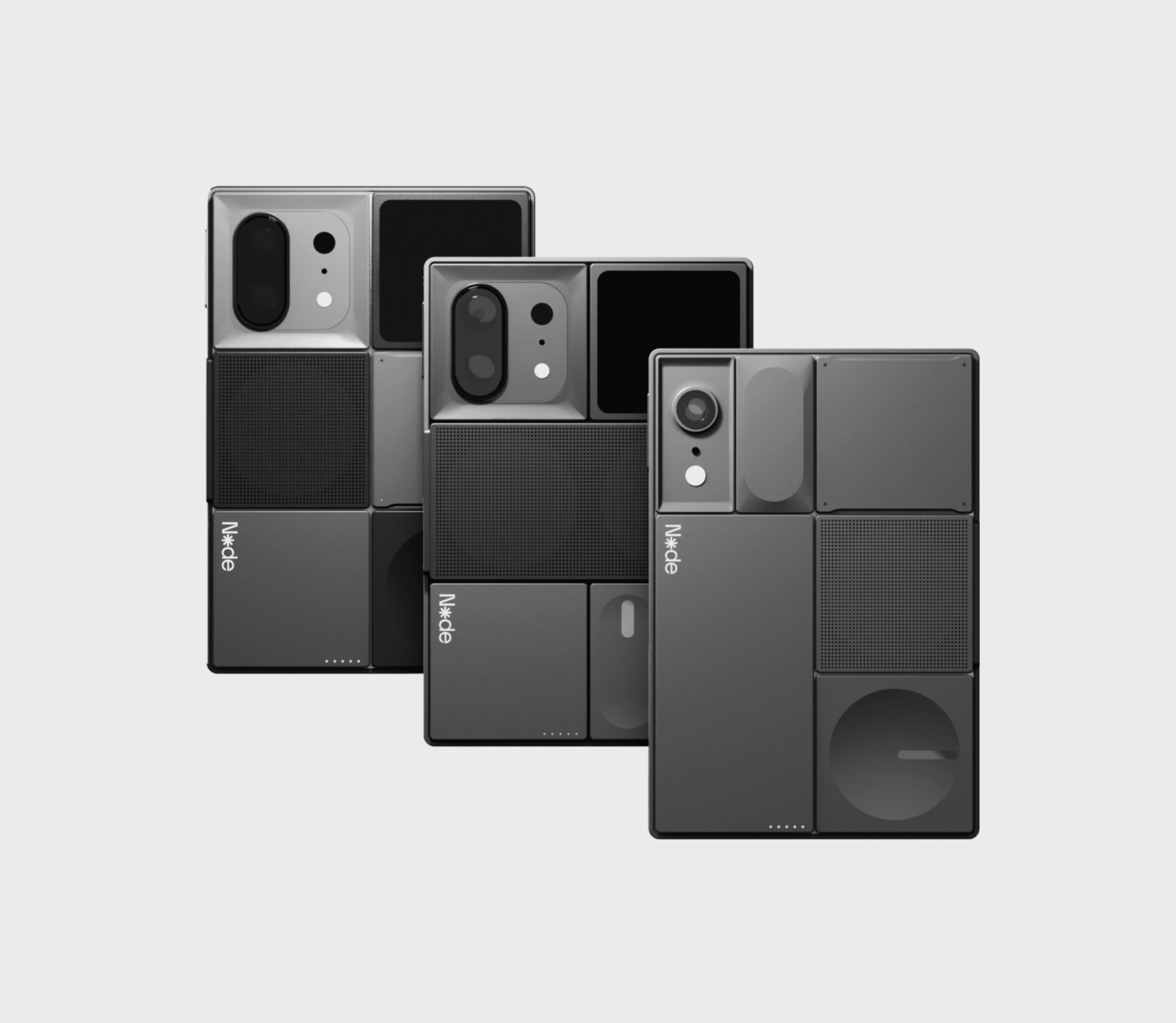
8th Jan, 2025

What Does a Perfect On-Page SEO Landing Page Structure Look Like?

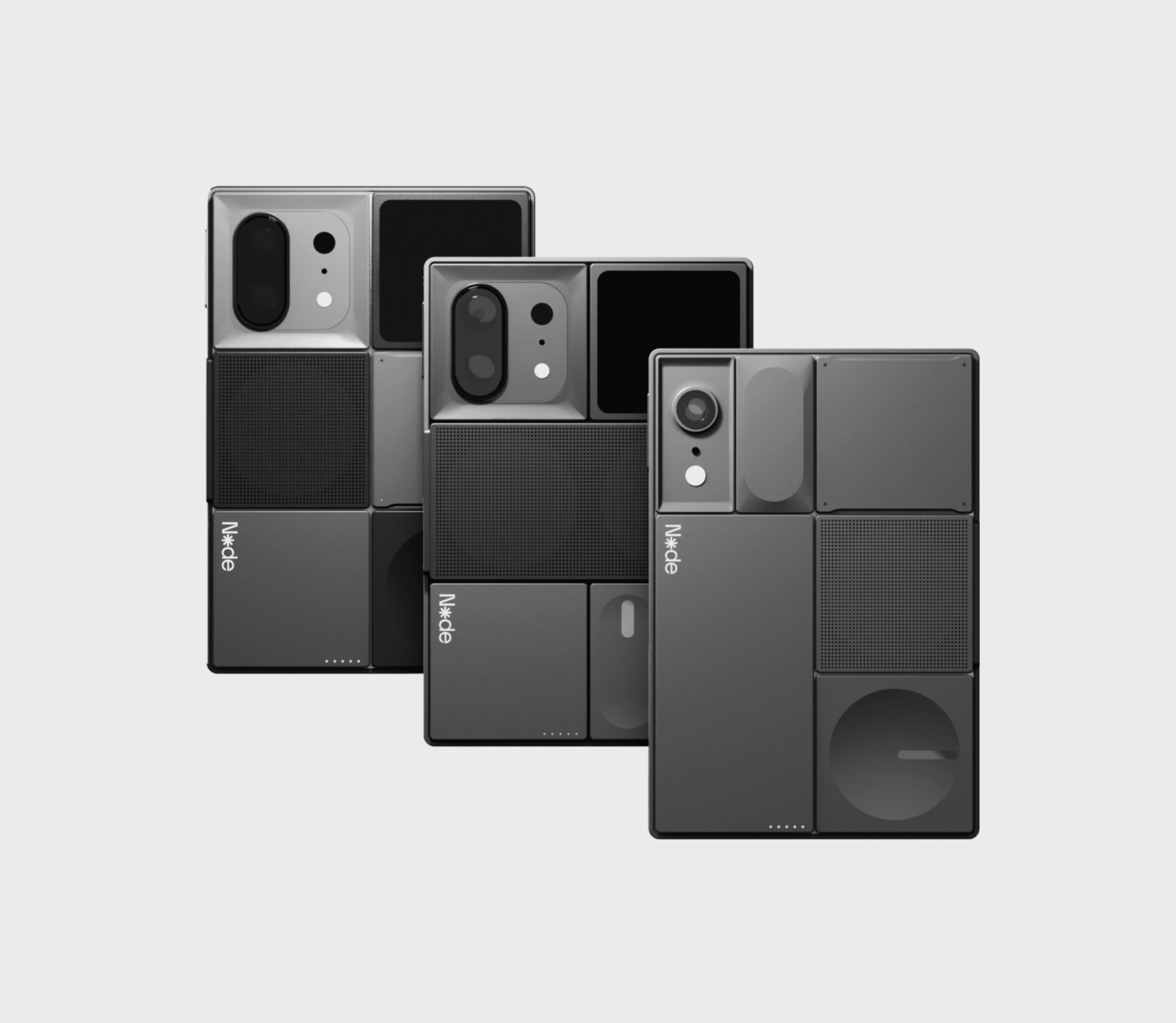
8th Jan, 2025

What Does a Perfect On-Page SEO Landing Page Structure Look Like?

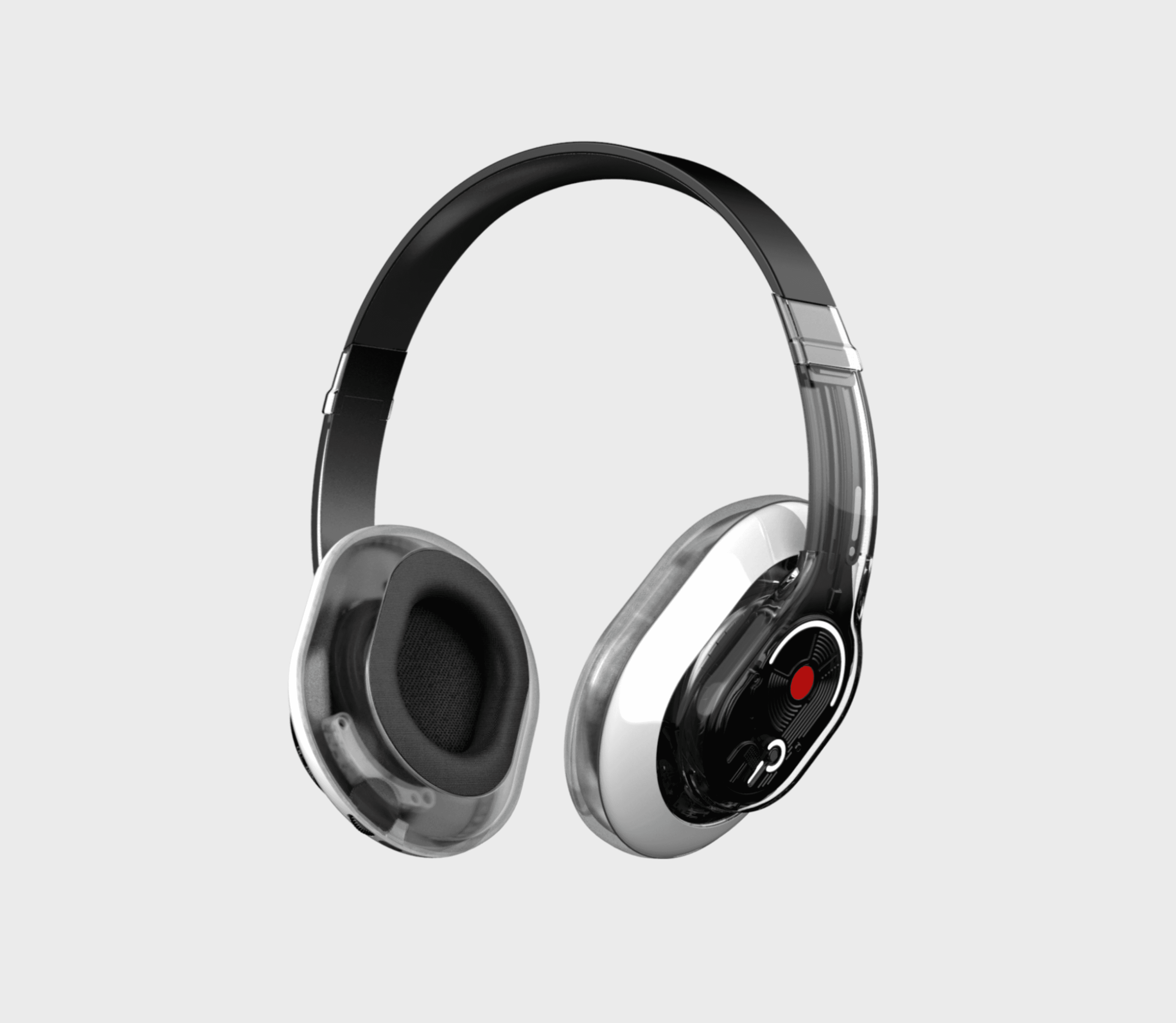
10th Jan, 2025

Boost Your Web Presence in 5 Minutes with Framer Templates

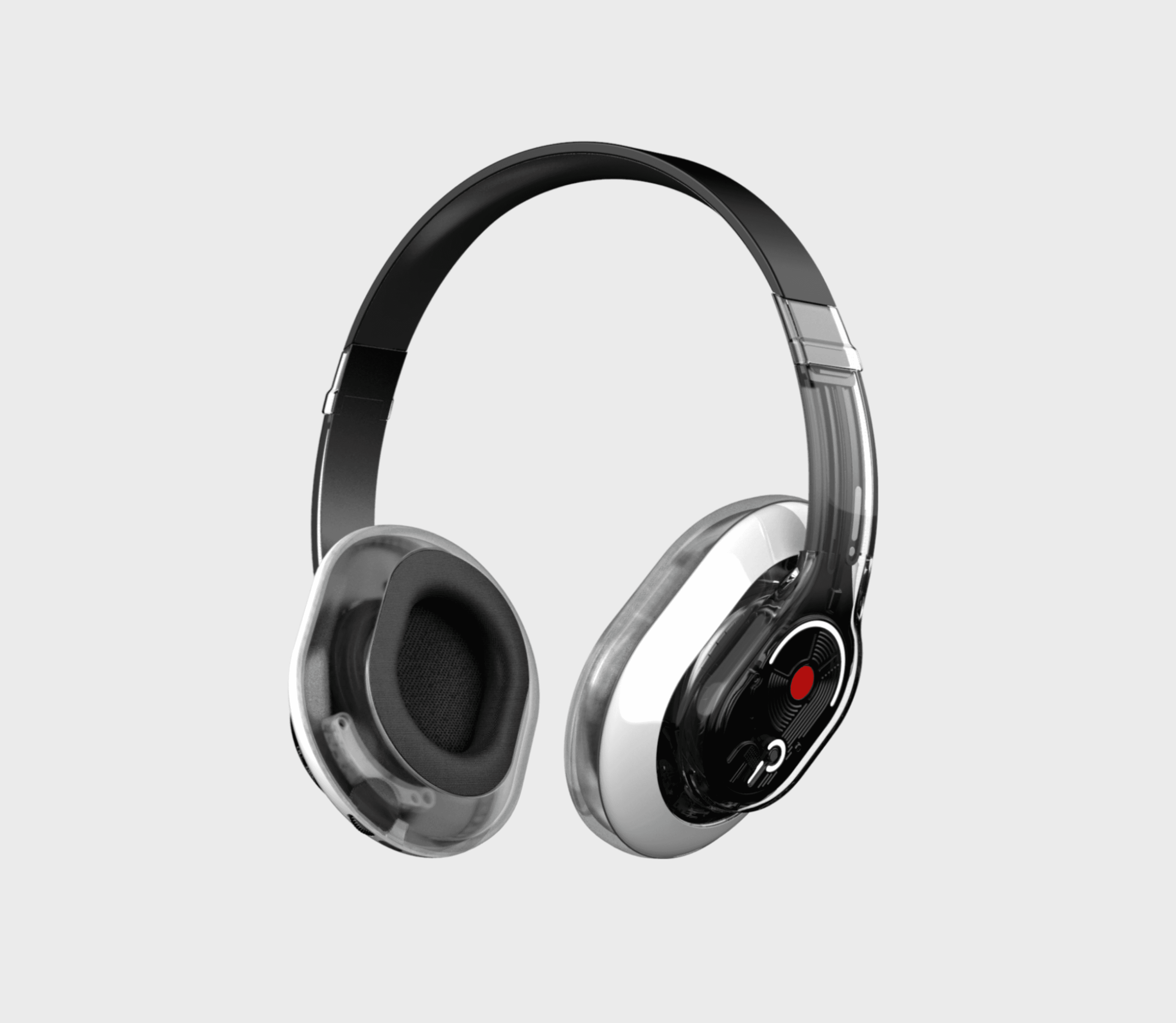
10th Jan, 2025

Boost Your Web Presence in 5 Minutes with Framer Templates

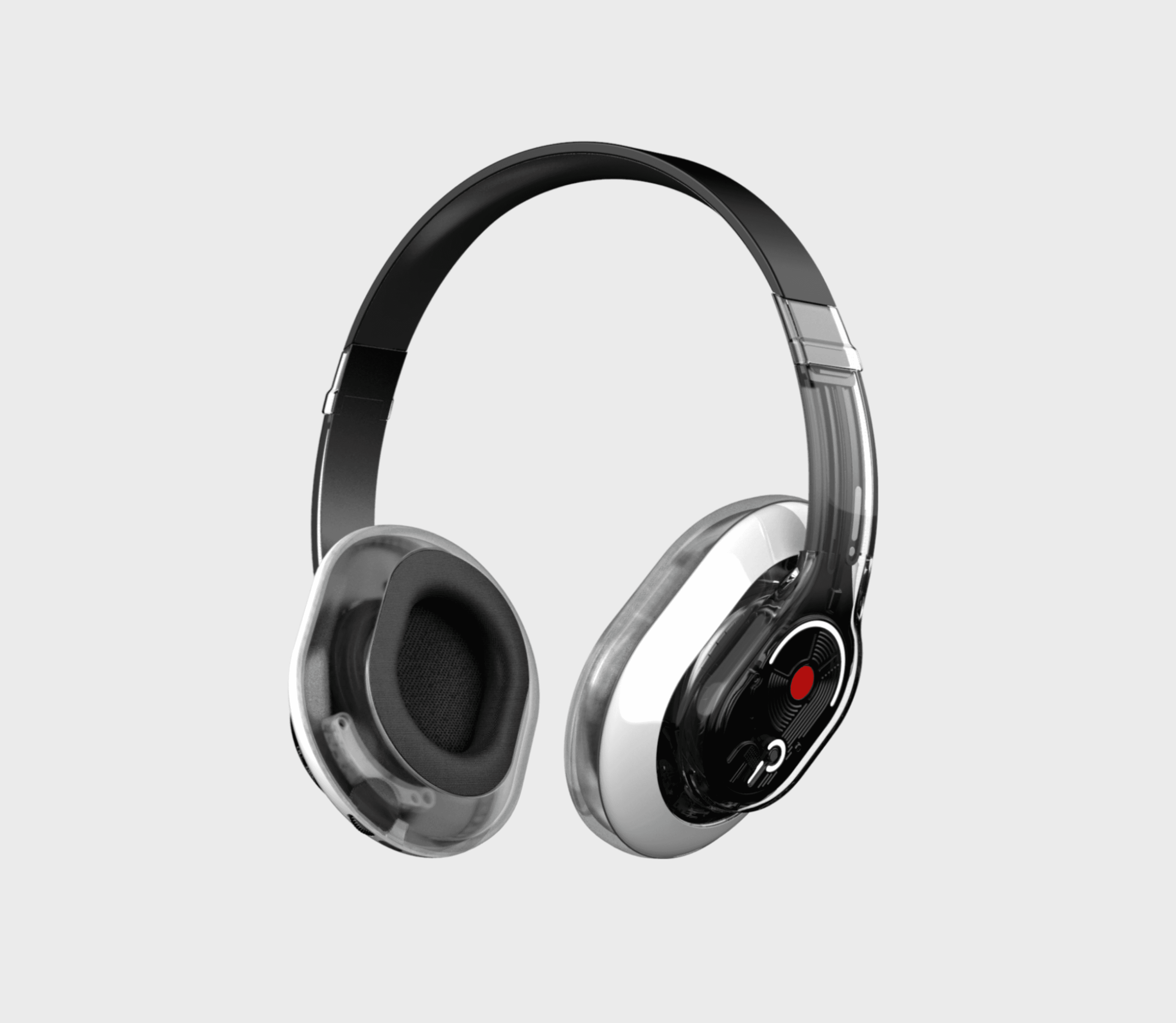
10th Jan, 2025

Boost Your Web Presence in 5 Minutes with Framer Templates

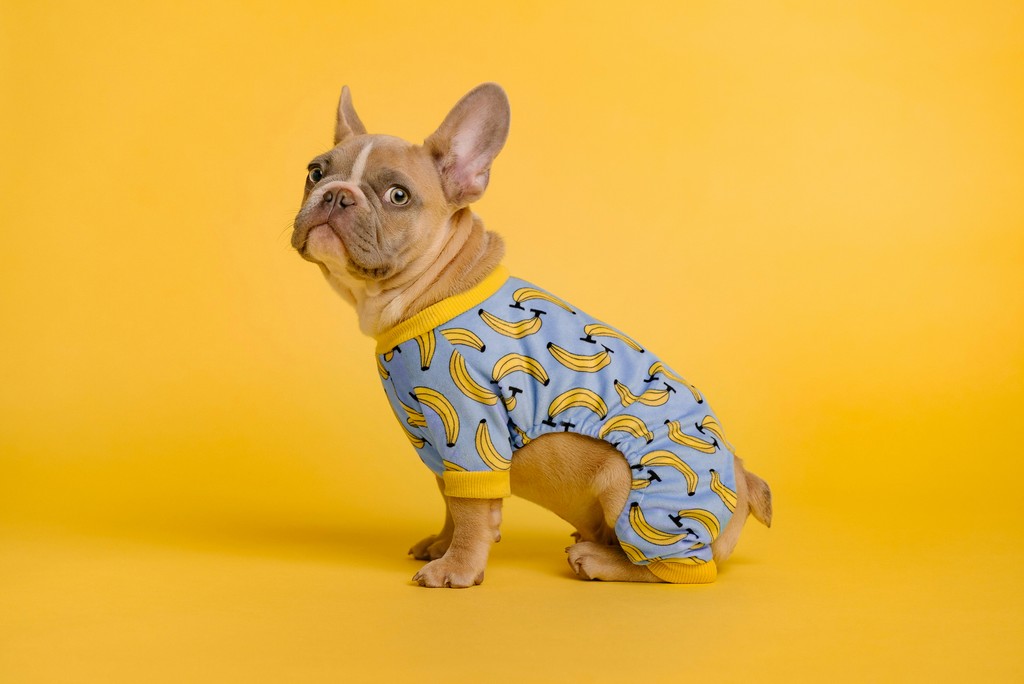
5th Jan, 2025

What Are the Best Website Analytics Tools to Track and Optimize Your Portfolio Site?

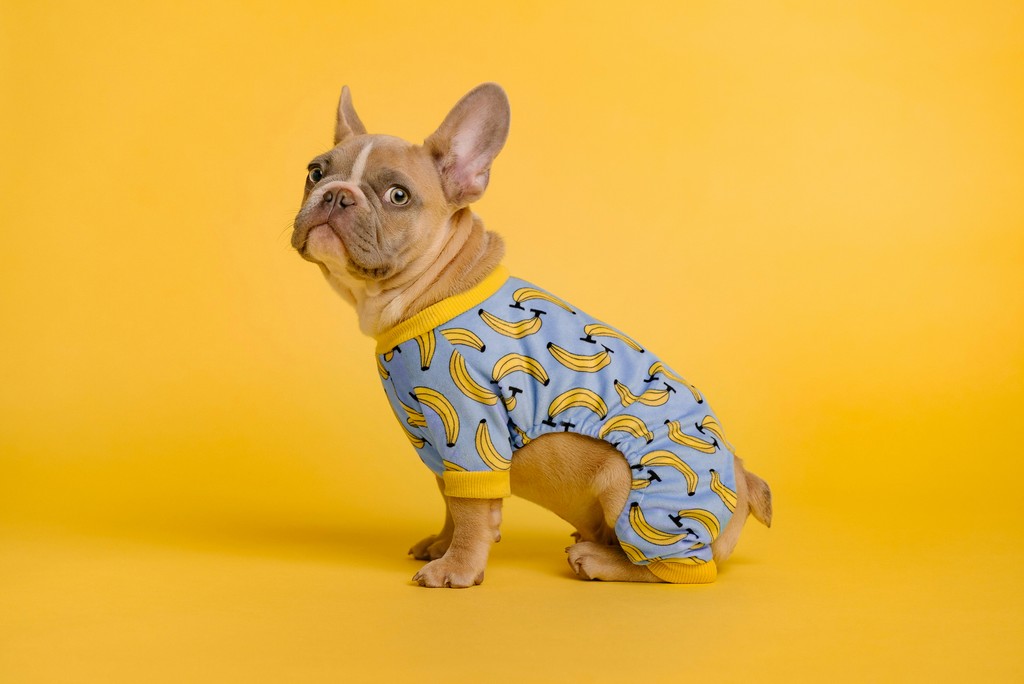
5th Jan, 2025

What Are the Best Website Analytics Tools to Track and Optimize Your Portfolio Site?

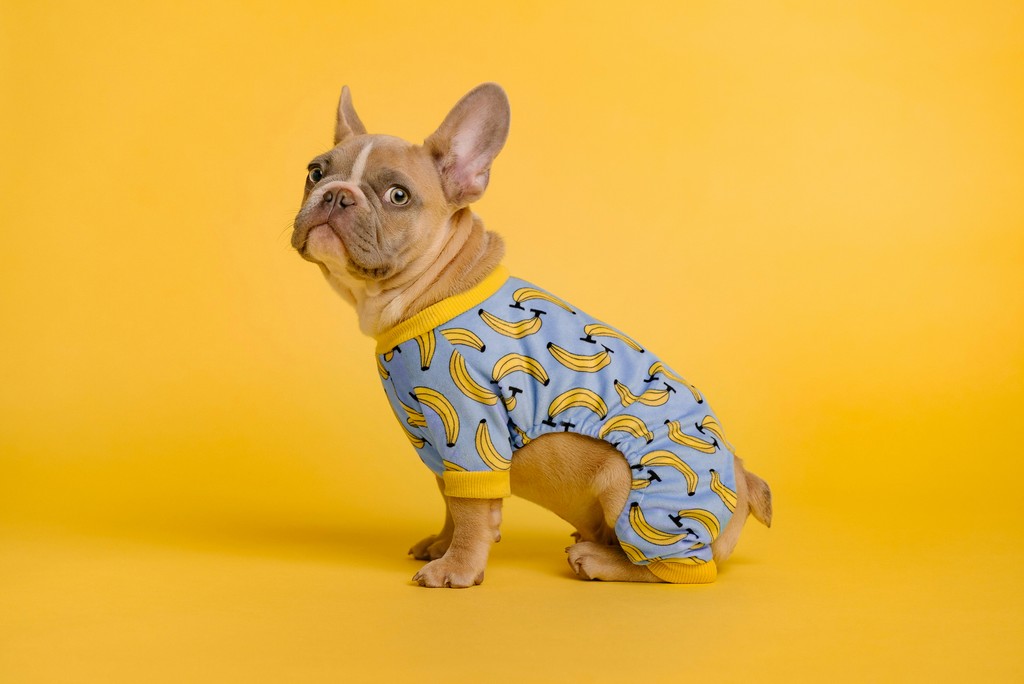
5th Jan, 2025

What Are the Best Website Analytics Tools to Track and Optimize Your Portfolio Site?
Frequently
Frequently asked
Frequently
Asked Questions
Questions
Asked Question
What makes 6thsensesites different from other web agencies?
What is Framer, and why do you use it?
What types of businesses do you work with?
What is a UI/UX audit, and why is it important?
What is your project process?
What is the difference between SEO and AEO?
Do you offer website maintenance after launch?
What makes 6thsensesites different from other web agencies?
What is Framer, and why do you use it?
What types of businesses do you work with?
What is a UI/UX audit, and why is it important?
What is your project process?
What is the difference between SEO and AEO?
Do you offer website maintenance after launch?
What makes 6thsensesites different from other web agencies?
What is Framer, and why do you use it?
What types of businesses do you work with?
What is a UI/UX audit, and why is it important?
What is your project process?
What is the difference between SEO and AEO?
Do you offer website maintenance after launch?